|
|
GameX R6 - Core Redesign Project
GameX is currently undergoing a major
redesign. A special team of Part 2 students from the Game Design Initiative
at Cornell University is developing projects that contribute to the
expansion of GameX.
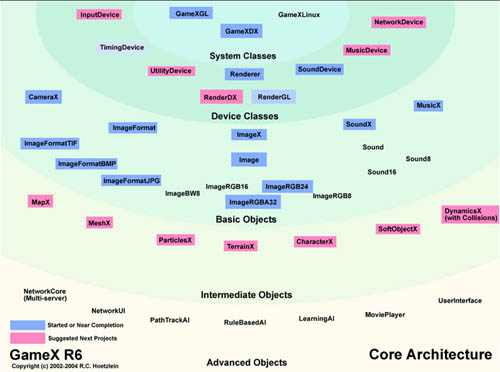
(click for large, printable image)
The new GameX architecture (R6) is divided into layers.
Much of the code for the System and Device layers already exists, or
is currently in development. Projects that are available include some
classes on these levels and any objects on the Intermediate and Advanced
object levels. In addition, there are many possible projects that aren't
shown in this diagram (for example, Raycasting or BSP Trees for efficient
rendering) that you might wish to design and contribute. A more detailed
description of some possible projects is below.
The GameX design layers are as follows:
I) System classes - System classes support
the 'single interface' feature unique to GameX. They are used directly
by the game developer to call functions that display graphics, play
sounds and music, or get user input. The System objects are 'singleton'
classes, meaning that only one instance of that class will exist in
any program or game. Most of the functionality of a System class is
multiply-inherited from several Device classes. Since each System class
can inherit from a different set of Device clases, there can be a System
classes for each combination of platform and low-level rendering system.
GameXGL supports OpenGL rendering under Windows, GameXDX supports DirectX
rendering under Windows, GameXLinuxGL supports OpenGL rendering under
X-Windows on Linux. There are several System classes, but only one will
exist in any given program or game.
II) Device classes - Device classes support
specific functionality on a specific platform or system. There are Device
classes to handle rendering in DirectX (the RenderDX class), rendering
in OpenGL (the RenderGL class), sound output under Windows (the SoundDevice
class), and music output under Windows (the MusicDevice class). Other
Device classes support game timing control, user input and utility functions
to aid the game developer. Each Device classes provides a set of functions
(interface) that the game developer should find easy-to-use. In addition,
the interfaces for Device classes should also be cross-platform, so
that the mechanism of the Device is handled internally. For example,
the Renderer base class provides basic functions for graphics output,
such as: GameX.DrawImage (&myimg). Since GameX is inherited from
a Renderer Device class, the user can request the GameX object to handle
the request directly. However, the Renderer class is itself a base class
for the RenderDX and RenderGL Device classes. The RenderDX and RenderGL
classes both support the DrawImage function, with the same parameters
and inputs, but they handle the requests differently. One renders using
DirectX and the other using OpenGL. In this way, Devices can be mixed
and matched to support a variety of platforms.
III) Basic objects - Basic objects support
the storage and manipulation of common game objects such as images,
sounds and music clips. The Basic objects actually maintain the data
for these objects, while the Device classes support their output. For
example, an ImageX object is passed into the DrawImage function of the
Renderer Device class. The Basic objects are sent to devices so that
they can be displayed or output. The Renderer Device knows what an ImageX
object is because both are a part of the GameX core. The game developer
(user) can create as many Basic objects as they need to in order to
store images and sounds. Basic objects should provide functions for
creating, manipulating and loading these objects from disk. For example,
the ImageX class provides functions to create an image of a specific
size (ImageX.Size), clear the image with a specific color (ImageX.Fill),
or load an image from disk (ImageX.Load).
IV) Intermediate objects - Intermediate
objects are similar to Basic objects except that they might depend on
Basic objects being available. An ImageX Basic object can exist by itself,
it simply maintains pixel data for the image. However, the MeshX object,
which represents a solid, textured 3D object using polygons must store
both the polygon geometry that describe the object, but also maintain
the images that define the textures applied to that object. Since a
MeshX object may maintain and store several ImageX objects for textures,
it is an Intermediate object.
While there are only a few Basic objects, there can be a huge number
of Intermediate objects. The Intermediate objects shown above define
a few of the features that game developers need. These include:
MapX - A two-dimensional array of images that can be loaded
and rendered. In many games, the background map has certain features
- mountains, streams, trees, etc. These might be stored as a set of
ImageX objects, where the user can specify the images for each of these.
The map itself could be stored as a 2D array of values, where the value
indicates which image to render for that grid space. Challenges include
loading and saving maps, providing a tool for creating maps, and figuring
out how to render the map through the Render Device class.
MeshX - Represents a solid, textured, three-dimensional object.
Challenges here include methods of representing surfaces composed of
polygons, the connectivity between polygons (edges and faces), loading
and saving objects, the application of ImageX objects as textures, and
rendering the MeshX object through the Renderer Device class.
ParticleX - This class represents a three-dimensional particle
system. Challenges include representing the position, velocity, and
acceleration of 3D particles, determining how they will be colored and
rendered, simulating their motion, and applying external forces to the
particles. (See the ParticleDemoR5.04 for a demonstration of a particle
system)
TerrainX - A terrain object would represent a there-dimensional
landscape. Landscapes could be represented with the MeshX object, but
there are other additional challenges to representing terrain. These
challenges include alternative representations (may be better to represent
the height of a terrain as an image, then construct 3D geometry based
on this image rather than storing the 3D geometry explicitly), dealing
with the scale problems of terrain, and providing convenient functions
to the user (like a function that given an X/Y point on the map, returns
the height of the terrain where a character can be placed to appear
on the surface).
CharacterX - A character object represents the three-dimensional
jointed articulation of a human (or other) character. Challenges include
representing jointed hierarchies, forward kinematics, loading and saving
character 'skeletons', providing scripts to animate motions like walking,
running, and jumping. A advanced topic in this area is inverse kinematics,
the problem of determining the angular rotation of each joint based
on a specified goal at a terminal point (if I move my hand to pick up
a glass, how do the angles of my shoulder and elbow change over that
time)
SoftObjectX - A soft object represents a deformable, or "soft",
three-dimensional object. Some examples include jello, a mattress, or
a cloth. Soft objects are usually represented with a particle system,
in which the particles are connected by a spring system of interconnections.
The spring system keeps the particles structurally connected while also
allowing them to deform and move around. Challenges include representing
the soft-object structure (particles and springs), representing the
geometry of the object (surface polygons that can be rendered), allowing
the user to apply external forces, and making sure the simulation is
robust and efficient.
FluidX - Representation of three-dimensional fluids. The challenges
associated with fluids include representing the geometry of the fluid
(should fluids be maintained as particles or as a three-dimensional
grid of small fluid volumes), the structure of the fluid (velocity and
pressure of the fluid at each point), determining how to render the
fluid, and computing effects on the fluid such as an object dropping
into it.
DynamicsX - A dynamics class would represent the physics and
motion of rigid objects. Unlike particle systems, which deal with the
forces on infinitesimal points, rigid-body dynamics extends this to
objects that have arbitrary shape. One way this might be accomplished
is for the DynamicsX class to maintain a list of MeshX objects that
participate in a dynamic simulation. The dynamics class would then have
the challenges of determining when two objects collided, computing the
forces and rotational torque on each object as a result of a collision,
and simulating and updating the position and rotation of each object
when it is moving freely.
V) Advanced objects - Advanced objects
are similar to Intermediate objects (they depend on Basic objects being
available), but may require the development of several classes to support
a single feature. Advanced objects may require more time and effort
than Intermediate objects. Some Advanced projects include the following:
NetworkingUI - This Advanced project deals with how a user connects
to an on-line game. Typically, the user would start a 'multiplayer'
panel in the game, which would locate a server or allow you to specify
one then permit the user to connect to and participate in a game. Challenges
include locating and listing available servers, communicating with the
server to request a client connection, and accepting commands from the
game server.
Multi-Server Networking - This Advanced project would develop
the client, server, manager relationships that would be needed to support
massively multiplayer games. Unlike smaller networked games, where there
is a single server with multiple client, massively multiplayer games
might have several coordinated servers where each one handles a small
portion of the entire game world. Challenges include developing a network
topology, dividing the game world among servers, handling individual
clients and passing clients between servers, and considering a managing
server that maintains a consistent gaming experiences across multiple
servers.
Path Tracking AI - Path tracking deals with the artificial intelligence
problem of finding the simplest path for an object from its current
point to a goal while avoiding any obstacles along the way. This problem
is solved many different ways depending on how objects and space are
represented. For example, on a regular 2D grid (MapX), the solution
involves finding a path through grid squares. On three-dimensional terrain,
the solution might involve avoiding objects of different heights and
shapes while attempting to traverse to the goal.
Rule Based AI - Rule-based AI defines the behavior of intelligent
agents in a game based on a set of rules. Challenges include representing
the state (or knowledge) of an agent, representing the rules that agents
must following, determining when and how to apply rules to an agent,
and incorporating any additional constraints (eg. the agent cannot walk
through walls).
Learning AI - Learning AI is similar to Rule Based AI, except
that agents may have the potential to learn from the user or from their
previous behavior. This is a very new topic in computer game development.
Challenges include determining a learning strategy, generating and testing
hypotheses (possible new rules or behavior), evaluating current behavior
relative to the user, and avoiding unwanted behavior (eg. the agent
might learn that the best way to survive is to avoid the player altogether)
MoviePlayer - Industry-level computer games are beginning to
approach to technical and visual quality of film. In newer games, movie
sequences are often mixed with game play to contribute to the plot and
story of the game. This requires that the game have the ability to load
and play back movie clips. Challenges include supporting the various
compression/decompression (codec) techniques used to efficiently store
and playback movie clips, and developing functions that allow the user
to build or extract single images from a movie (eg. the user might which
to extract a single frame from a movie into an ImageX object for use
in the game itself)
UserInterface - In general, the appearance of a game will follow
a specific format: 2D Side Scrolling, 3D Orthogonal view, 2D Top Down
view, 3D First Person, 3D Third Person. However, in all of these cases,
there might be menus, buttons, and options that appear to the player.
A User-Interface typically consists of a set of generic functions that
support these features. Challenges include developing a set of objects
that support the behavior of menus, buttons, lists, and context menus,
interfacing with the mouse and keyboard to provide control over these
objects, and allowing the user to define a specific visual appearance
of the user interface while maintaining a general behavior.
|
|
|